Digest Cycles in Single Page Apps
Brendan Graetz
What is a Digest Cycle?
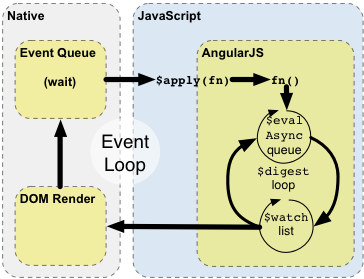
What is a Digest Cycle?
- Code that runs at an interval
- Typically used to aggregate expensive tasks
Where are they used?
- "Game Loop"
- An introduction
- An in-depth explanation
Where are they used?
- SPA frameworks
- AngularJS
- (coined the term)
- EmberJS
- (look for Ember.run and runLoop)
- Accessors vs. Dirty-checking
Where are they -not- used?
- BackboneJS
Why are they important in SPAs?
- Dirty checking
- Improve efficiency
- Aggregate DOM changes
- (render to buffer)
- Aggregate listeners
- Computed properties
(computed properties)
Computed properties allow you to treat a function like a property
App.Person = Ember.Object.extend({
bmi: function() {
var height = this.get('height');
return this.get('weight') / height / height;
}.property('weight', 'height')
});
Avoid in digest cycles
Too much work in each cycle
Avoid in digest cycles
Too much work in each cycle
var socket = io.connect();
return {
on: function (eventName, callback) {
socket.on(eventName, _.throttle(function () {
var args = arguments;
$rootScope.$apply(function () {
callback.apply(socket, args);
});
}, 500)); // limit to once every 500ms
}
// ...
};